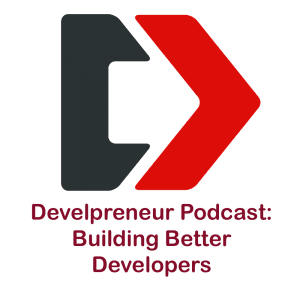
Timothy Stratton Interview Part 2 – The Value of Higher Education
Part two of our series of interviews with Timothy Stratton looks at his early years. He started with a focus on higher education. That worked well for him, but not directly because of his studies. We explore how the journey taught him more than the academic focus. A Little Background Timothy is a software development manager at XSolis with a strong development background. He has a master’s degree and a love for learning. We gave some details in part one, but dig deeper into how his career started and how those steps lead to success today. Higher Education Side Effects The value of a degree is often noted as coming from the work done more than the learning itself. Timothy... Read more